Testing ensures that an application works the way it is meant to, fulfills its purpose, and remains secure. Examples of testing frameworks include Python, Pytest, Robot framework, and Behave. In Python testing frameworks, some have specific capabilities and advantages over others. The Python framework language plays a very important role in functional testing, as they provide tools for code development, automated tests, code quality improvement, reliability, and effectiveness.
PyTest is one general testing framework most commonly used to perform unit and functional tests; it supports features such as fixtures, parameterization, and robust plugin support. The Robot Framework, conversely, is a keyword-driven test tool mainly used for acceptance testing and robotic process automation (RPA). Lastly, Behave extends BDD with natural language-based test case definitions. Through this analysis of frameworks, developers and testers can ascertain the most suitable tools for their applications to implement effective and versatile testing solutions.
The purpose of this guide is to provide a thorough understanding of each Python testing framework that will help professionals realize their full potential in real-world applications by utilizing machine learning models.
Understanding Python testing frameworks
Python test frameworks make testing application functionality straightforward, improving testing efficiency and automation. Key characteristics provided by the testing frameworks include test exploration, setup and teardown functions, reporting, and integration with tools. PyTest streamlines unit testing through an easy-to-use syntax and numerous plugin choices. The keyword-driven methodology of Robot Framework makes it ideal for acceptance testing since it enables non-programmers to set up tests.
By facilitating collaboration among developers and stakeholders through test descriptions expressed in a standard language, Behave facilitates behavior-driven development (BDD). The right framework is chosen based on the testing requirements, project complexity, and team collaboration. Utilizing Python testing frameworks improves software dependability and maintainability, guaranteeing strong applications.
Testing levels in Python
Testing in Python is organized into various levels to guarantee the thorough validation of applications. Python testing can be divided into various levels:
● Unit testing: This represents the most fundamental testing level, focusing on the evaluation of individual functions or methods independently. It guarantees that small, independent segments of code operate properly.
● Integration testing: This stage emphasizes testing the interaction among various modules or components. It guarantees that merged units operate correctly when brought together.
● System testing: In this phase, the complete application system undergoes testing as a unit. The aim is to confirm that the entire application fulfills the defined requirements.
● Acceptance testing: This final assessment stage is carried out to verify that the application satisfies the needs of the organization in question. Robot Framework and Behave are two examples of frameworks that help with acceptance testing.
Python’s testing ecosystem
The Python testing ecosystem allows for overall quality, fewer errors, and faster development by combining all the features necessary for the support of many testing frameworks. The Python testing environment was developed to support application testing while ensuring that reliability and maintainability are preserved within the coding scope. Unit testing, behavior-driven development, and BDD are examples of testing methods that are typically supported by the frameworks.
Unittest
The included unittest module offers a typical method to create and execute tests in Python. It adheres to the xUnit style, providing assertions, test identification, and setup/cleanup methods.
PyTest
PyTest is a robust and flexible testing framework that enhances unit testing with its wide plugin library, fixture support, parameterization features, and easy-to-understand syntax. It facilitates parallel testing, effective test execution, and smooth continuous integration and continuous delivery integration.
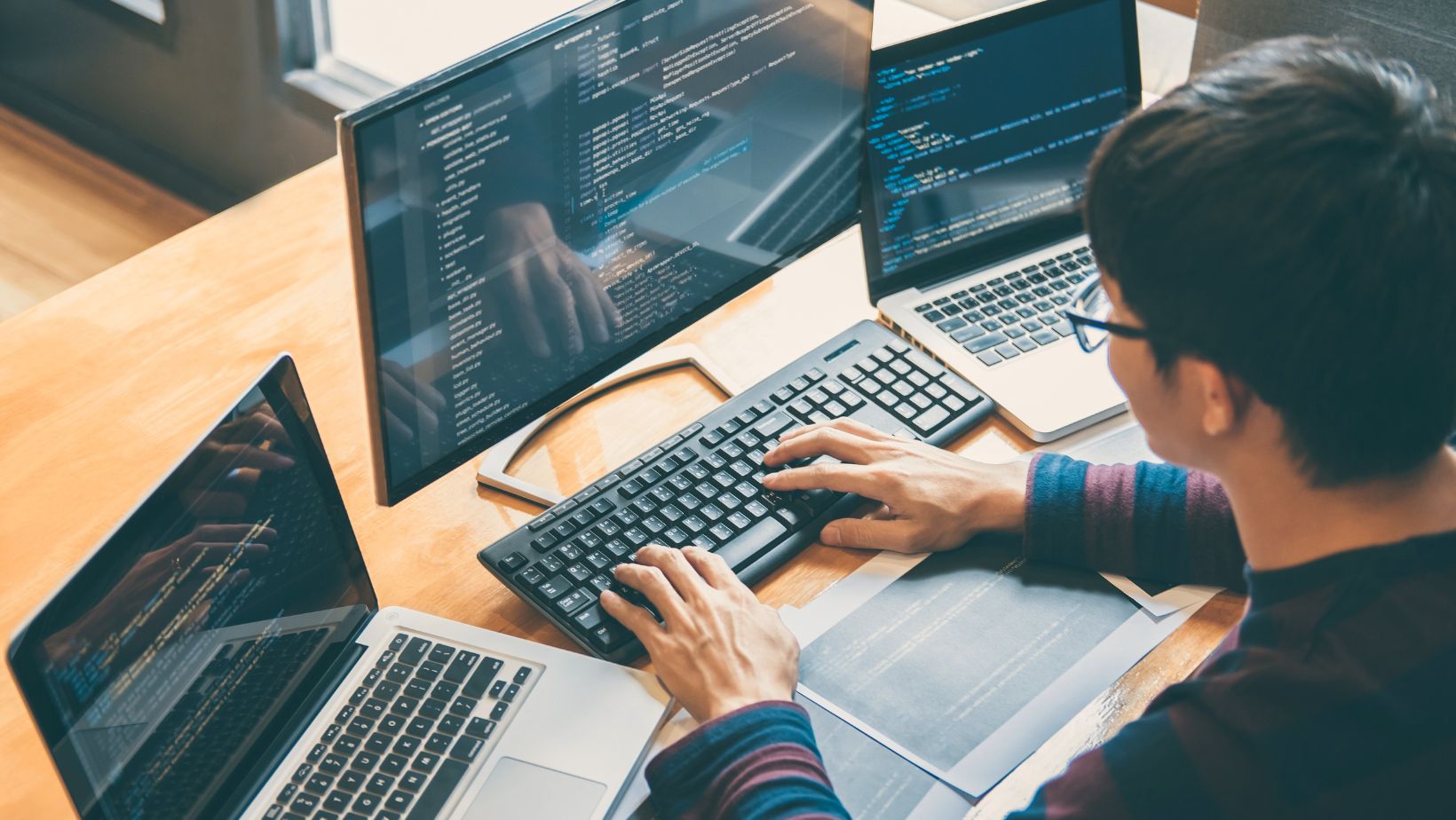
PyTest is an increasingly popular choice for both small and large projects. Since it offers high-level capabilities including fixture management, test discovery, and integrated reporting.
Because the framework does not require unnecessary code, tests done using pytest are much more condensed. The built-in capabilities of pytest aid in the automatic finding of test methods and modules.
Key features of PyTest
PyTest provides functionalities like:
● Simple and uncomplicated syntax: PyTest uses a simple and intelligible methodology that avoids unnecessary characteristics, producing tests that are easier to read and manage.
● Parameterized testing: Parameterized testing improves test coverage by allowing the same test to be run with different data sets through the use of the @pytest.mark.parametrize decorator.
● Test conclusion: It eliminates the need for particular test suite definitions by automatically identifying and running tests by naming standards.
● Setup and breakdown fixtures: This provides a strong framework for fixtures that allows for reusable scripts for setup and removal, reducing duplication and improving test flexibility.
● Assertions with comprehensive reporting: Utilizes Python’s built-in assert function and delivers precise failure messages along with improved debugging details.
Finest practices for using PyTest
To enhance the efficiency and efficacy of PyTest, adhere to these recommended practices:
● Employ fixtures for setup and teardown: Fixtures assist in handling test dependencies and the logic for setup and removal. Reusable components improve modularity and decrease repetitive code.
● Utilize parameterization: Utilize @pytest.mark.parametrize to run the identical test with different data sets, improving coverage and reducing code duplication.
● Arrange tests in an organized way: Keep tests in a tests/ folder and label test files with the test_ prefix to guarantee automatic detection.
● Utilize assertions wisely: Steer clear of intricate assertion logic. Utilize straightforward assert statements to enhance clarity and ease of debugging.
● Mark and skip tests: Utilize @pytest.mark.skip and @pytest.mark.xfail to manage test execution according to specific conditions.
Robot framework
A testing framework driven by keywords, mainly utilized for acceptance testing and robotic process automation (RPA). It allows non-technical developers to define test cases with easily readable keywords and can integrate with libraries such as Selenium for web automation tasks. Robot Framework is highly flexible, supporting the use of custom keywords, testing libraries, and external extensions. It also offers comprehensive test reports and logs for improved test analysis.
Key features of the robot Framework
The robot framework offers numerous key features that help in understanding keywords. They are:
● Extensively extensible: It accommodates custom keywords, user-created libraries, and plug-ins to enhance its features.
● Comprehensive test reporting: Produces detailed, easily analyzable logs and reports in HTML format, enhancing debugging efficiency.
● Vast library assistance: Includes pre-installed libraries and enables integration with external libraries such as Selenium, Appium, and Database libraries for thorough automation.
● Cross-platform Compatibility: Any operating system-based environment; thus, it can be used in a variety of testing environments.
● Keyword-Driven Testing: A beginner-friendly method that allows test construction through reusable keywords using a simple syntax, so it is yet another utility for users whose main expertise doesn’t lie within programming.
Finest practices for using Robot Frameworks
Below are some key techniques of robot frameworks:
● Employ descriptive keywords: Establish significant and reusable keywords to enhance test clarity and support.
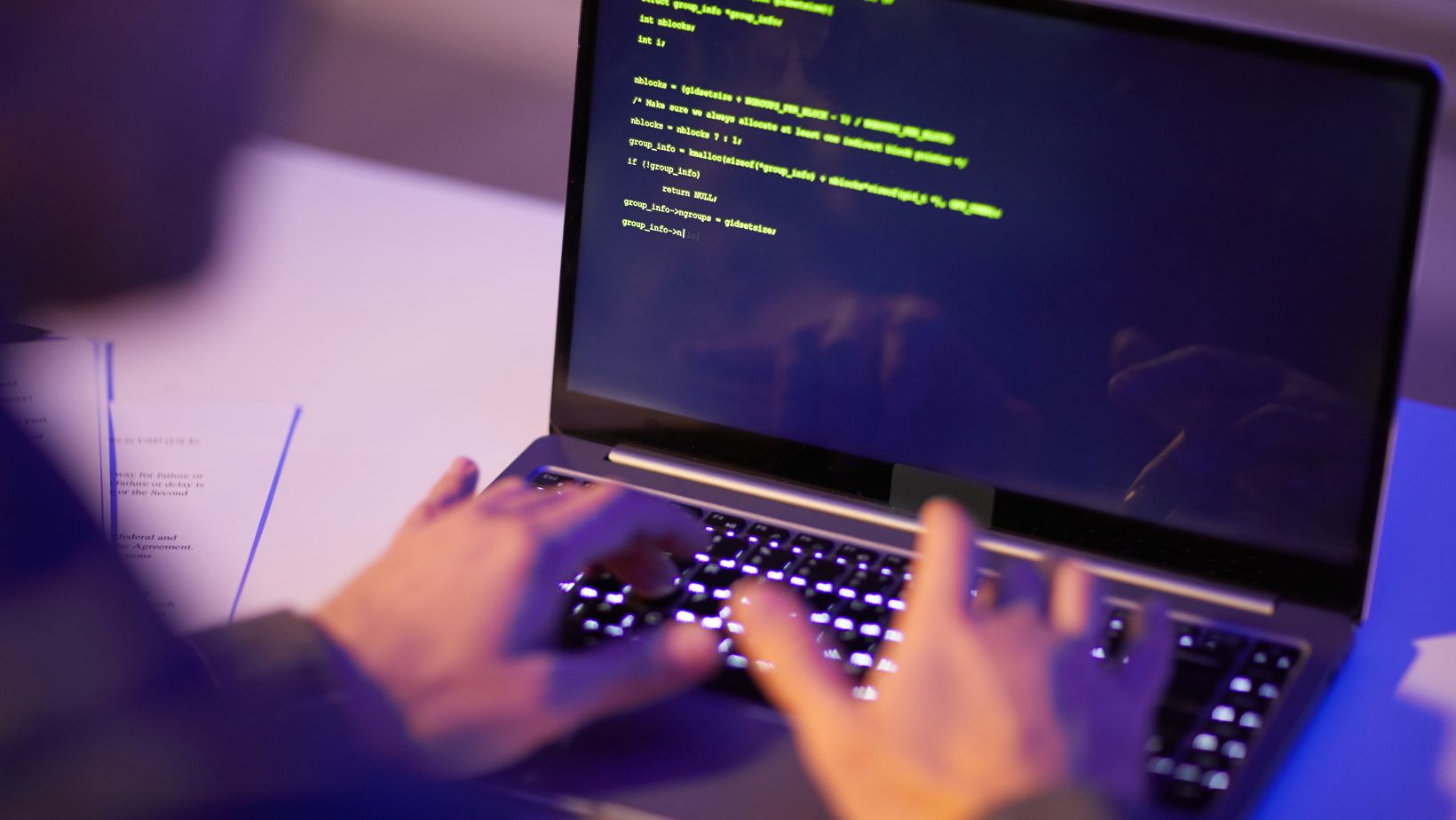
● Arrange test cases effectively: Categorize test cases into distinct test suites by utilizing directories and resource files to achieve modularity.
● Employ available and third-party libraries: Make use of Robot Framework’s built-in libraries while incorporating external libraries (such as Selenium, Requests) for improved capabilities.
● Utilize variables efficiently: Save reusable values in variables to enhance the adaptability of test cases and minimize repetition.
● Apply data-driven testing: Utilize test templates along with external data sources (CSV, Excel) to execute tests across various datasets.
● Enable parallel run: Cut down the test run time by running tests in parallel with tools like Pabot.
Behave
It is possible to write BDD tasks in Behave, as it allows developers, testers, and company stakeholders to communicate the testing scenarios in simple English using Gherkin. Behave makes it assurance for tests to be simple and easily understandable for stakeholders with little or no technical background. It is a necessary element for agile and BDD teams because it aids in defining steps, performing scenarios, and executing reusable test cases.
Key features of Behave
Below are some of the key features of Behave:
● Gherkin syntax for clarity: A structure deploying the Given-When-Then format model so both technical and non-technical participants can know the test scenarios.
● Behavior-driven development (BDD) assistance: These support collaboration between developers, testers, and organizational analysts by applying familiar terms in explaining test scenarios.
● Collaboration with additional testing tools: Can operate in conjunction with Selenium and various automation tools for complete testing approaches.
● Basic and adaptable structure: Allows for custom Python functions to enhance the framework’s features.
● Enables tag support for test execution control: Facilitates the selective running of test cases using tags, enhancing test oversight.
● Comprehensive test reports and logging: Produces easily understandable execution reports to enhance debugging and analysis.
● Simple integration with CI/CD pipelines: It can be effortlessly incorporated into automation pipelines, guaranteeing ongoing validation of application functionalities.
Finest Practices for Behave
Behavior-driven development (BDD) using Behave promotes teamwork and transparency in creating tests. Adhering to these best practices guarantees successful test execution:
● Draft clear and succinct feature files: Employ straightforward, easy-to-understand language when outlining scenarios to enhance the accessibility of tests for all stakeholders.
● Repurpose step definitions: Eliminate duplication by developing reusable step definitions for frequent actions found in various scenarios.
● Arrange tests into rational frameworks: Keep distinct folders for features, steps, and auxiliary files to enhance maintainability.
● Utilize tags for test selection: Employ tags to classify and selectively execute test scenarios, enhancing test performance.
● Utilize data tables for parameterization: Streamline test cases by employing tables to manage various test inputs effectively.
● Integrate with automation frameworks: Combine Behave with Selenium or API testing frameworks for comprehensive full-stack testing. Both the local Selenium installation and the online Selenium grid provided by cloud testing platforms such as LambdaTest can be used to run the implemented tests.
One can run Python automated tests with Selenium at scale across several operating systems and browsers with LambdaTest, an AI-powered test execution and orchestration platform. For a more detailed study on what is selenium? You can reach out to the LambdaTest platform.
The platform provides a cloud-based Selenium grid that can run PyTest and BDD test scenarios on a cloud of over 3000+ browsers and operating systems configurations simultaneously. Integrating Python frameworks with LambdaTest gives testers the power of cloud-based cross-browser testing, which is crucial for ensuring that their web application works well across various environments and devices.
LambdaTest enables comprehensive coverage and facilitates real-time monitoring of test runs, quickly identifies issues, and helps users refine their scripts efficiently. It has several advantages, but the primary ones are faster test execution, cheaper maintenance, and lower expenses.
Conclusion
The testing ecosystem of Python provides a variety of frameworks designed for different testing requirements. PyTest offers a versatile and effective method for unit testing through robust fixtures and plugins. Robot Framework is perfect for keyword-driven and acceptance testing, offering a wide range of libraries for automation. Behave enhances teamwork via BDD, rendering tests clearer and easier to comprehend.
By utilizing these frameworks efficiently, teams can enhance software reliability, optimize automation, and guarantee smooth integration with CI/CD pipelines. Grasping their strengths and effective methods allows developers and testers to create strong, scalable, and maintainable test suites for delivering high-quality applications.
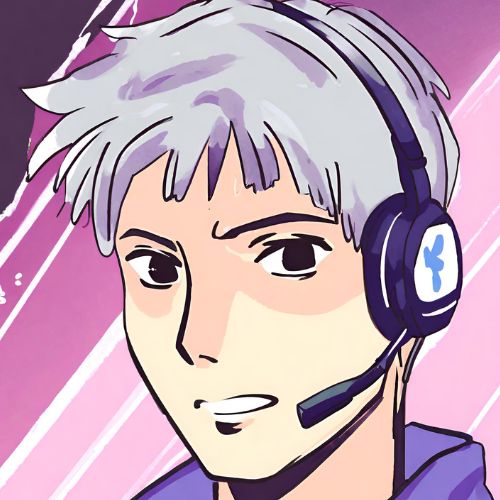